Jquery Tutorials
- What is JQuery
- DOM Document Object Model
- JQuery Syntax
- Jquery Selector
- Get & Set Form value
- jQuery - Attributes
- Attribute Methods
- jQuery - DOM Manipulation
- JQuery Events
- JQuery Effects
- JQuery Html/Css
- jQuery Insert Content
- Auto Hide Div
- JQuery noConflict()
- JQuery Form Validation
- Form Validation
- Login Form Validation
- Jquery Fadeout message
- Modal popup
- Jquery Ajax Forms
- Dependent Dropdown
- Autocomplete Country jquery ajax
- Dynamic Content Load using jQuery AJAX
- Dynamic star rating jQuery AJAX
- Drag and Drop Image Upload
- show Hide Elements By click on checkbox
- How to Add class in jQuery
- calculate discount in jQuery
- Calculate GST by input value in text box
- check Password strength in jQuery
- Count Remaining Character
- onClick Checkbox check all
- password match or not
- DataTable
- Date Picker
- Multiselect Dropdown with Checkbox
- Add Dynamic Input Field (Add More Input Field)
- submit button disable after one click
- Show hide password in Password textbox using checkbox
- Put value in the text field
- Set Data and Attributes
Customer Say
Jquery Autocomplete Country list.
Autocomplete feature is used to provide auto suggestion for users while entering input. In this tutorial, we are going to suggest country names for the users based on the keyword they entered into the input field by using jQuery AJAX.
File name : index.php
<html>
<head>
<TITLE>jQuery AJAX Autocomplete - Country Example</TITLE>
<head>
<style>
body{width:610px;}
.frmSearch {border: 1px solid #F0F0F0;background-color:#C8EEFD;margin: 2px 0px;padding:40px;}
#country-list{float:left;list-style:none;margin:0;padding:0;width:190px;}
#country-list li{padding: 10px; background:#FAFAFA;border-bottom:#F0F0F0 1px solid;}
#country-list li:hover{background:#F0F0F0;}
#search-box{padding: 10px;border: #F0F0F0 1px solid;}
</style>
<script src="https://code.jquery.com/jquery-2.1.1.min.js" type="text/javascript"></script>
<script>
$(document).ready(function(){
$("#search-box").keyup(function(){
$.ajax({
type: "POST",
url: "readCountry.php",
data:'keyword='+$(this).val(),
beforeSend: function(){
$("#search-box").css("background","#FFF url(LoaderIcon.gif) no-repeat 165px");
},
success: function(data){
$("#suggesstion-box").show();
$("#suggesstion-box").html(data);
$("#search-box").css("background","#FFF");
}
});
});
});
function selectCountry(val) {
$("#search-box").val(val);
$("#suggesstion-box").hide();
}
</script>
</head>
<body>
<div class="frmSearch">
<input type="text" id="search-box" placeholder="Country Name" />
<div id="suggesstion-box"></div>
</div>
</body>
</html>
File name : dbcontroller.php
<?php
class DBController {
private $host = "localhost";
private $user = "root";
private $password = "";
private $database = "phppot_examples";
function __construct() {
$conn = $this->connectDB();
if(!empty($conn)) {
$this->selectDB($conn);
}
}
function connectDB() {
$conn = mysql_connect($this->host,$this->user,$this->password);
return $conn;
}
function selectDB($conn) {
mysql_select_db($this->database,$conn);
}
function runQuery($query) {
$result = mysql_query($query);
while($row=mysql_fetch_assoc($result)) {
$resultset[] = $row;
}
if(!empty($resultset))
return $resultset;
}
function numRows($query) {
$result = mysql_query($query);
$rowcount = mysql_num_rows($result);
return $rowcount;
}
}
?>
File name : readCountry.php
<?php
require_once("dbcontroller.php");
$db_handle = new DBController();
if(!empty($_POST["keyword"])) {
$query ="SELECT * FROM country WHERE country_name like '" . $_POST["keyword"] . "%' ORDER BY country_name LIMIT 0,6";
$result = $db_handle->runQuery($query);
if(!empty($result)) {
?>
<ul id="country-list">
<?php
foreach($result as $country) {
?>
<li onClick="selectCountry('<?php echo $country["country_name"]; ?>');"><?php echo $country["country_name"]; ?></li>
<?php } ?>
</ul>
<?php } } ?>
Output :-
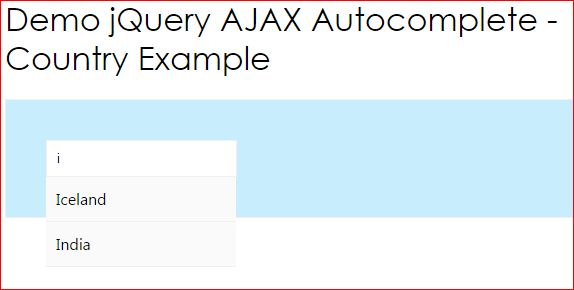
Output :-
Jquery Autocomplete Country list.
Download Jquery Autocomplete Country list zip files
demo link :- phppot.com