Jquery Tutorials
- What is JQuery
- DOM Document Object Model
- JQuery Syntax
- Jquery Selector
- Get & Set Form value
- jQuery - Attributes
- Attribute Methods
- jQuery - DOM Manipulation
- JQuery Events
- JQuery Effects
- JQuery Html/Css
- jQuery Insert Content
- Auto Hide Div
- JQuery noConflict()
- JQuery Form Validation
- Form Validation
- Login Form Validation
- Jquery Fadeout message
- Modal popup
- Jquery Ajax Forms
- Dependent Dropdown
- Autocomplete Country jquery ajax
- Dynamic Content Load using jQuery AJAX
- Dynamic star rating jQuery AJAX
- Drag and Drop Image Upload
- show Hide Elements By click on checkbox
- How to Add class in jQuery
- calculate discount in jQuery
- Calculate GST by input value in text box
- check Password strength in jQuery
- Count Remaining Character
- onClick Checkbox check all
- password match or not
- DataTable
- Date Picker
- Multiselect Dropdown with Checkbox
- Add Dynamic Input Field (Add More Input Field)
- submit button disable after one click
- Show hide password in Password textbox using checkbox
- Put value in the text field
- Set Data and Attributes
Customer Say
JQuery Form Validation.
Enable or Disable Submit Button
how to enable or disable form submit button based on the form validation result.
File name : index.php
<html>
<head>
<title>Enable Disable Submit Button Based on Validation</title>
<style>
#btn-submit{padding: 10px 20px;background: #555;border: 0;color: #FFF;display:inline-block;margin-top:20px;cursor: pointer;}
#btn-submit:disabled{padding: 10px 20px;background: #CCC;border: 0;color: #FFF;display:inline-block;margin-top:20px;cursor: no-drop;}
.validation-error {color:#FF0000;}
.input-control{padding:10px;}
.input-group{margin-top:10px;}
</style>
</head>
<body>
<h1>Enable Disable Submit Button Based on Validation</h1>
<form id="frm" method="post">
<div class="input-group">Name <span class="name-validation validation-error"></span></div>
<div>
<input type="text" name="name" id="name" class="input-control" onblur="validate()" />
</div>
<div class="input-group">Email <span class="email-validation validation-error"></span></div>
<div>
<input type="text" name="email" id="email" class="input-control" onblur="validate()" />
</div>
<div>
<button type="submit" name="btn-submit" id="btn-submit" disabled="disabled">Submit</button>
</div>
</form>
<script src="http://code.jquery.com/jquery-1.10.2.js"></script>
<script>
function validate() {
var valid = true;
valid = checkEmpty($("#name"));
valid = valid && checkEmail($("#email"));
$("#btn-submit").attr("disabled",true);
if(valid) {
$("#btn-submit").attr("disabled",false);
}
}
function checkEmpty(obj) {
var name = $(obj).attr("name");
$("."+name+"-validation").html("");
$(obj).css("border","");
if($(obj).val() == "") {
$(obj).css("border","#FF0000 1px solid");
$("."+name+"-validation").html("Required");
return false;
}
return true;
}
function checkEmail(obj) {
var result = true;
var name = $(obj).attr("name");
$("."+name+"-validation").html("");
$(obj).css("border","");
result = checkEmpty(obj);
if(!result) {
$(obj).css("border","#FF0000 1px solid");
$("."+name+"-validation").html("Required");
return false;
}
var email_regex = /^([a-zA-Z0-9_.+-])+\@(([a-zA-Z0-9-])+\.)+([a-zA-Z0-9]{2,3})+$/;
result = email_regex.test($(obj).val());
if(!result) {
$(obj).css("border","#FF0000 1px solid");
$("."+name+"-validation").html("Invalid");
return false;
}
return result;
}
</script>
</body>
</html>
Output :-
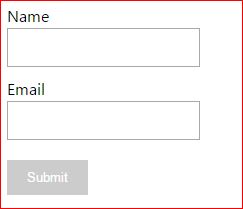
Invalid Data in Form Field Restrict Typing using jQuery
File name : index.php
<html>
<head>
<title>Restrict Typing Invalid Data in Form Field using jQuery</title>
<style>
#btn-submit{padding: 10px 20px;background: #555;border: 0;color: #FFF;display:inline-block;margin-top:20px;cursor: pointer;}
#btn-submit:disabled{padding: 10px 20px;background: #CCC;border: 0;color: #FFF;display:inline-block;margin-top:20px;cursor: no-drop;}
.validation-error {color:#FF0000;}
.input-control{padding:10px;}
.input-group{margin-top:10px;}
</style>
</head>
<body>
<h1>Restrict Typing Invalid Data in Form Field using jQuery</h1>
<form id="frm" method="post">
<div class="input-group">Name <span class="name-validation validation-error"></span></div>
<div>
<input type="text" name="name" id="name" class="input-control" onblur="validate()" />
</div>
<div class="input-group">Phone <span class="phone-validation validation-error"></span></div>
<div>
<input type="text" name="phone" id="phone" class="input-control" onblur="validate()" />
</div>
<div>
<button type="submit" name="btn-submit" id="btn-submit" disabled="disabled">Submit</button>
</div>
</form>
<script src="http://code.jquery.com/jquery-1.10.2.js"></script>
<script>
$(document).ready(function() {
$('#name').on('keypress', function(key) {
if((key.charCode < 97 || key.charCode > 122) && (key.charCode < 65 || key.charCode > 90) && (key.charCode != 45)) {
return false;
}
});
$('#phone').on('keypress', function(key) {
if(key.charCode < 48 || key.charCode > 57) {
return false;
}
});
});
function validate() {
var valid = true;
valid = checkEmpty($("#name"));
valid = valid && checkEmpty($("#phone"));
$("#btn-submit").attr("disabled",true);
if(valid) {
$("#btn-submit").attr("disabled",false);
}
}
function checkEmpty(obj) {
var name = $(obj).attr("name");
$("."+name+"-validation").html("");
$(obj).css("border","");
if($(obj).val() == "") {
$(obj).css("border","#FF0000 1px solid");
$("."+name+"-validation").html("Required");
return false;
}
return true;
}
</script>
</body>
</html>
Output :-
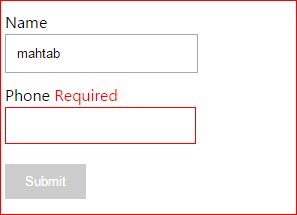
jQuery Form Validation with Tooltip
File name : index.php
<link rel="stylesheet" href="//code.jquery.com/ui/1.11.4/themes/smoothness/jquery-ui.css">
<style>
body{width:610px;}
#frmContact {border-top:#F0F0F0 2px solid;background:#FAF8F8;padding:10px;}
#frmContact div{margin-bottom: 15px}
#frmContact div label{margin-left: 5px}
.demoInputBox{padding:10px; border:#F0F0F0 1px solid; border-radius:4px;}
.btnAction{background-color:#2FC332;border:0;padding:10px 40px;color:#FFF;border:#F0F0F0 1px solid; border-radius:4px;}
.invalid{border:#FF0000 1px solid;}
</style>
<style>
.ui-tooltip {padding: 10px;color: #333;font-size: 12px;background: #FFACAC ;}
</style>
<script src="https://code.jquery.com/jquery-2.1.1.min.js" type="text/javascript"></script>
<script src="//code.jquery.com/ui/1.11.4/jquery-ui.js"></script>
<script>
function sendContact() {
var valid;
valid = validateContact();
if(valid) {
jQuery.ajax({
url: "contact_mail.php",
data:'userName='+$("#userName").val()+'&userEmail='+$("#userEmail").val()+'&subject='+$("#subject").val()+'&content='+$(content).val(),
type: "POST",
success:function(data){
$("#mail-status").html(data);
},
error:function (){}
});
}
}
function validateContact() {
var valid = true;
$("#frmContact input[required=true], #frmContact textarea[required=true]").each(function(){
$(this).removeClass('invalid');
$(this).attr('title','');
if(!$(this).val()){
$(this).addClass('invalid');
$(this).attr('title','This field is required');
valid = false;
}
if($(this).attr("type")=="email" && !$(this).val().match(/^([\w-\.]+@([\w-]+\.)+[\w-]{2,4})?$/)){
$(this).addClass('invalid');
$(this).attr('title','Enter valid email');
valid = false;
}
});
return valid;
}
$(function() {
$( document ).tooltip({
position: {my: "left top", at: "right top"},
items: "input[required=true], textarea[required=true]",
content: function() { return $(this).attr( "title" ); }
});
});
</script>
<div id="frmContact">
<div id="mail-status"></div>
<label style="padding-top:20px;">Name</label>
<div>
<input type="text" name="userName" id="userName" class="demoInputBox" required="true">
</div>
<label>Email</label>
<div>
<input type="email" name="userEmail" id="userEmail" class="demoInputBox" required="true">
</div>
<label>Subject</label>
<div>
<input type="text" name="subject" id="subject" class="demoInputBox" required="true">
</div>
<label>Content</label>
<div>
<textarea name="content" id="content" class="demoInputBox" cols="60" rows="6" required="true"></textarea>
</div>
<div>
<button name="submit" class="btnAction" onClick="sendContact();">Send</button>
</div>
</div>
Output :-
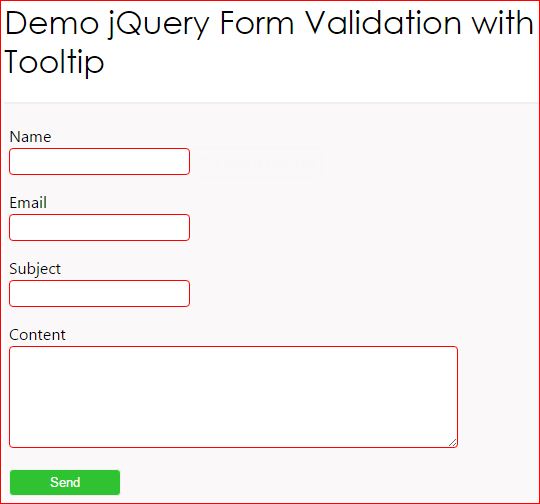
File Size Validation using jQuery
File name : index.php
<html>
<head>
<style>
body{width:610;}
#frmFile {border-top:#F0F0F0 2px solid;background:#FAF8F8;padding:10px;}
.demoInputBox{padding:10px; border:#F0F0F0 1px solid; border-radius:4px;background-color:#FFF;}
#file_error{color: #FF0000;}
#btnSubmit{background-color:#2FC332;border:0;padding:10px 40px; margin:15px 0px; color:#FFF;border:#F0F0F0 1px solid; border-radius:4px;}
</style>
<script src="http://code.jquery.com/jquery-2.1.1.js"></script>
<script>
function validate() {
$("#file_error").html("");
$(".demoInputBox").css("border-color","#F0F0F0");
var file_size = $('#file')[0].files[0].size;
if(file_size>2097152) {
$("#file_error").html("File size is greater than 2MB");
$(".demoInputBox").css("border-color","#FF0000");
return false;
}
return true;
}
</script>
</head>
<body>
<form name="frmFile" id="frmFile" method="post" action="" onSubmit="return validate();">
<div><input type="file" name="file" id="file" class="demoInputBox" /> <span id="file_error"></span></div>
<div><input type="submit" id="btnSubmit" value="Upload"/></div>
</form>
</body>
</html>
Character Count using jQuery
File name : index.php
<html>
<head>
<title>Twitter Style Remaining Character Count using jQuery</title>
<style>
body{width:610px;}
#text-content{padding:10px; border:#F0F0F0 1px solid; border-radius:4px;background-color:#FFF;}
#text-content:focus{outline: 0;}
#character-count{color: #A0A0A0;padding: 15px 0px;}
</style>
<script src="https://code.jquery.com/jquery-2.1.1.min.js" type="text/javascript"></script>
<script>
function count_remaining_character() {
var max_length = 150;
var character_entered = $('#text-content').val().length;
var character_remaining = max_length - character_entered;
$('#character-count').html(character_remaining);
if(max_length < character_entered) {
$('#character-count').css('color','#FF0000');
} else {
$('#character-count').css('color','#A0A0A0');
}
}
</script>
</head>
</body>
<textarea id="text-content" cols="80" rows="4" onKeyup="count_remaining_character()"></textarea>
<div id="character-count" align="right">150</div>
</html>
Output :-
Jquery form validation
File name : index.php
<html>
<head>
<title>JQUERY Form Validation</title>
<link rel="stylesheet" href="http://code.jquery.com/ui/1.10.4/themes/smoothness/jquery-ui.css">
<script src="http://code.jquery.com/jquery-1.9.1.js"></script>
<script src="http://code.jquery.com/ui/1.10.4/jquery-ui.js"></script>
<!-- jquery validation plugin //-->
<script type="text/javascript" src="http://ajax.microsoft.com/ajax/jquery.validate/1.7/jquery.validate.js"></script>
<link rel="stylesheet" href="style.css" type="text/css" />
<style type="text/css">
.hidden{
display:none;
}
</style>
<script type="text/javascript">
$(document).ready(function()
{
$("#form_register").validate(
{
rules:{
'name':{
required: true,
minlength: 1
},
'username':{
required: true,
minlength: 3,
username_regex: true,
remote:{
url: "validatorAJAX.php",
type: "post"
}
},
'email':{
required: true,
email: true,
remote:{
url: "validatorAJAX.php",
type: "post"
}
},
'web':{
required: true,
url: true
},
'date':{
required: true,
date: true
},
'pass1':{
required: true,
minlength: 8
},
'pass2':{
equalTo: '#reg_pass1'
},
'gender':{
required:true,
}
},
messages:{
'name':{
required: "The name field is mandatory!",
minlength: "Choose a username of at least 1 letters!",
},
'username':{
required: "The username field is mandatory!",
minlength: "Choose a username of at least 4 letters!",
username_regex: "You have used invalid characters. Are permitted only letters numbers!",
remote: "The username is already in use by another user!"
},
'email':{
required: "The Email is required!",
email: "Please enter a valid email address!",
remote: "The email is already in use by another user!"
},
'web':{
required: "The Web Address is required!"
},
'pass1':{
required: "The password field is mandatory!",
minlength: "Please enter a password at least 8 characters!"
},
'pass2':{
equalTo: "The two passwords do not match!"
},
'gender':{
required: "must select gender"
},
}
});
});
</script>
</head>
<body>
<form action="" method="post" id="form_register">
<legend>Registration<legend>
<table width="50%">
<tr>
<td><label for="reg_name">Name*</label></td>
<td><input type="text" name="name" id="reg_name" /><br/></td>
</tr>
<tr>
<td><label for="reg_user">Username*</label></td>
<td><input type="text" name="username" id="reg_user" /><br/></td>
</tr>
<tr>
<td><label for="reg_email">Email*</label></td>
<td><input type="text" name="email" id="reg_email" /><br/></td>
</tr>
<tr>
<td><label for="reg_web">Website*</label></td>
<td><input type="text" name="web" id="reg_web" /><br/></td>
</tr>
<tr>
<td><label for="reg_date">Date of Birth*</label></td>
<td><input type="text" name="date" id="reg_date" /><br/></td>
</tr>
<tr>
<td><label for="reg_pass1">Password*</label></td>
<td><input type="password" name="pass1" id="reg_pass1" /><br/></td>
</tr>
<tr>
<td><label for="reg_pass1">Confirm Password*</label></td>
<td><input type="password" name="pass2" id="reg_pass1" /><br/></td>
</tr>
<tr>
<td><label for="reg_gender">Gender*</label></td>
<td>
<input type="radio" id="radio" name="gender" value="Female">Female
<input type="radio" id="radio" name="gender" value="Male">Male</td>
</tr>
<tr>
<td><input type="submit" name="register" value="Registraion" id="reg_submit" /></td>
<td><input type="reset" name="Cancel" value="Cancel" id="reg_reset" /></td>
</tr>
</table>
</form>
</body>
</html>
Output :-
File name : index.php
Output :-
File name : index.php
Output :-
File name : index.php
Output :-
File name : index.php
Output :-
File name : index.php
Output :-
File name : index.php
Output :-
File name : index.php
Output :-
File name : index.php
Output :-
File name : index.php