Laravel Tutorials
- What is laravel
- Laravel Installation
- Directory Structure
- htaccess
- Remove public from url
- Artisan Command
- Laravel Configuration
- Routing Configuration
- Namespaces
- Request
- Response
- Controller
- Model
- User Authentication
- Multi User Authentication
- Database Seeding
- Database
- Database Query
- ORM
- One-to-One Relationship
- One-to-Many Relationship
- Many to Many Eloquent Relationship
- Has One Through
- Has Many Through
- Querying Relations
- Middleware
- Laravel Views
- Blade Views
- Print data on view page
- Get Site URL
- Get URL Segment
- Get images from Storage folder
- Clear cache
- Form Class not found
- Flash Message in laravel
- Redirections
- path
- CRUD Projerct
- CRUD in Laravel
- CRUD progran
- Laravel Validation
- Jquery Validation
- Cookie
- Session
- Email Send in laravel
- File uploading
- CSRF Protection
- Helper in Laravel
- Helper Functions
- Guzzle Http Client
- Paypal Payment Gatway Integration
- Cron Job in laravel
- Flash message
- path
- Errors Handling
- Date Format
- Date Format Validation
- Display Image on View Page
- Update User Status using toggle button
- Delete Multiple Records using Checkbox in Laravel?
- Confirmation Before Delete Record
- Delete image from storage
- Remove/Trim Empty & Whitespace From Input Requests
- Block IP Addresses from Accessing Website
- How to Disable New User Registration in Laravel
- Redirect HTTP To HTTPS Using Laravel Middleware
- CKEditor
- slug generate unique
- Prevent Browser's Back Button After Logout
- Datatable dunamically
- encrypt & Decript
- Download File
- Rest API
- Shopping Cart
- Shopping Cart Example
- Dynamic Category-Subcategory Menu
- Ajax Search
- Interview Question
- laravel Tutorilal link
- laravel Tutorilal
Important Links
Laravel Configuration.
Basic Configuration :-
Environment Configuration :-
Environment variables are those which provide a list of web services to your web application. All the environment variables are declared in the .env file which includes the parameters required for initializing the configuration. By default, the .env file includes following parameters −
APP_ENV = local
APP_DEBUG = true
APP_KEY = base64:ZPt2wmKE/X4eEhrzJU6XX4R93rCwYG8E2f8QUA7kGK8 =
APP_URL = http://localhost
DB_CONNECTION = mysql
DB_HOST = 127.0.0.1
DB_PORT = 3306
DB_DATABASE = homestead
DB_USERNAME = homestead
DB_PASSWORD = secret
CACHE_DRIVER = file
SESSION_DRIVER = file
QUEUE_DRIVER = sync
REDIS_HOST = 127.0.0.1
REDIS_PASSWORD = null
REDIS_PORT = 6379
MAIL_DRIVER = smtp
MAIL_HOST = mailtrap.ioMAIL_PORT = 2525
MAIL_USERNAME = null
MAIL_PASSWORD = null
MAIL_ENCRYPTION = null
Important Points :-
While working with basic configuration files of Laravel, the following points are to be noted −
Retrieval of Environment Variables:-
All the environment variables declared in the .env file can be accessed by env-helper functions which will call the respective parameter. These variables are also listed into $_ENV global variable whenever application receives a request from the user end. You can access the environment variable as shown below −
'env' => env('APP_ENV', 'production'),
env-helper functions are called in the app.php file included in the config folder. The above given example is calling for the basic local parameter.
Accessing Configuration Values :-
You can easily access the configuration values anywhere in the application using the global config helper function. In case if the configuration values are not initialized, default values are returned.
For example, to set the default time zone, the following code is used −
config(['app.timezone' => 'Asia/Kolkata']);
Caching of Configuration:-
To increase the performance and to boost the web application, it is important to cache all the configuration values. The command for caching the configuration values is −
config:cache
c:/xampp/htdocs/itechxpert>php artisan config:cache
Maintenance Mode :-
Sometimes you may need to update some configuration values or perform maintenance on your website. In such cases, keeping it in maintenance mode, makes it easier for you. Such web applications which are kept in maintenance mode, throw an exception namely MaintenanceModeException with a status code of 503. You can enable the maintenance mode on your Laravel web application using the following command −
c:/xampp/htdocs/itechxpert>php artisan down
Once you finish working on updates and other maintenance, you can disable the maintenance mode on your web application using following command −
c:/xampp/htdocs/itechxpert>php artisan up
Database Configuration :-
The database of your application can be configured from config/database.php file. You can set configuration parameters that can be used by different databases and you can also set the default one to use.
'mysql' => [
'driver' => 'mysql',
'host' => env('DB_HOST', 'localhost'),
'port' => env('DB_PORT', '3306'),
'database' => env('DB_DATABASE', 'forge'),
'username' => env('DB_USERNAME', 'forge'),
'password' => env('DB_PASSWORD', ''),
'charset' => 'utf8',
'collation' => 'utf8_unicode_ci',
'prefix' => '',
'strict' => false,
'engine' => null,
],
Naming the Application :-
The App Directory, by default, is namespaced under App. To rename it, you can execute the following command and rename the namespace.
php artisan app:name <name-of-your-application>
Replace the <name-of-your-application> with the new name of your application that you want to give.
Maintenance Mode
We need to modify our website on a regular basis. The website needs to be put on maintenance mode for this. Laravel has made this job easier. There are two artisan commands which are used to start and stop the maintenance mode which are described below.
Start Maintenance Mode
To start the maintenance mode, simply execute the following command.
php artisan down
After successful execution, you will receive the following output −
Output :-
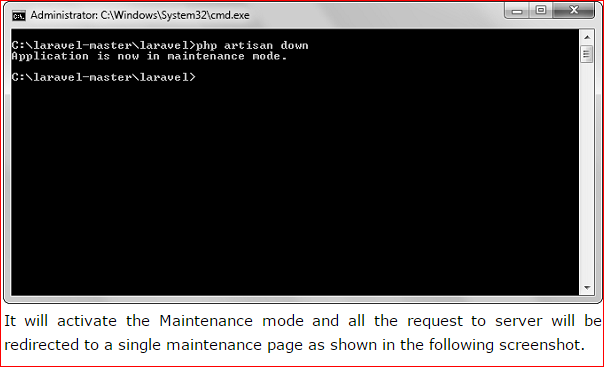
Stop Maintenance Mode
After making changes to your website and to start it again, execute the following command.
php artisan up
After successful execution, you will receive the following output −
Environment Variable Type
In laravel database configuration .env files allow every variable to pass through it as a string. Therefore some reserved values have been generated to facilitate you to return a broader range of types from the env() function:
If you require to define an environment variable including a value which incorporates spaces, you may do so by embedding the value in double quotes. A below-mentioned example will clear your doubts.
APP_NAME="My Application"
Retrieving Environment Configuration
The entire variables that are placed or listed in this file will be loaded inside the $_ENV PHP super-global when your application acquires a request. Though, you may utilize the env helper to recover or retrieve values from these variables in your configuration files.
‘debug’ => env(‘APP_DEBUG’, false),
The second value as you can see is a value passed to the “env” function is the “default value.” which will be utilized if no environment variable endures for the addressed key.
.htaccess file
File name : .htaccess
copy the .htaccess content from public directory htaccess file. and paste the root .htaccess file
<IfModule mod_rewrite.c>
<IfModule mod_negotiation.c>
Options -MultiViews -Indexes
</IfModule>
RewriteEngine On
# Handle Authorization Header
RewriteCond %{HTTP:Authorization} .
RewriteRule .* - [E=HTTP_AUTHORIZATION:%{HTTP:Authorization}]
# Redirect Trailing Slashes If Not A Folder...
RewriteCond %{REQUEST_FILENAME} !-d
RewriteCond %{REQUEST_URI} (.+)/$
RewriteRule ^ %1 [L,R=301]
# Handle Front Controller...
RewriteCond %{REQUEST_FILENAME} !-d
RewriteCond %{REQUEST_FILENAME} !-f
RewriteRule ^ index.php [L]
</IfModule>
Rename the server.php file to index.php
File name : index.php
Rename the server.php file to index.php
assets file
Directory name : public
All assets file store into public directory. such as All css, Js, images etc.
Set The .env file
File name : index.php
DB_DATABASE=mylara
DB_USERNAME=root
DB_PASSWORD=
collective not found
File name : index.php
[Symfony\Component\Debug\Exception\FatalErrorException]
Class 'Collective\Html\HtmlServiceProvider' not found
then, go on composer
c:/xampp/htdocs/mylara> composer require laravelcollective/html
composer dump-autoload
Then run:
composer update