Laravel Tutorials
- What is laravel
- Laravel Installation
- Directory Structure
- htaccess
- Remove public from url
- Artisan Command
- Laravel Configuration
- Routing Configuration
- Namespaces
- Request
- Response
- Controller
- Model
- User Authentication
- Multi User Authentication
- Database Seeding
- Database
- Database Query
- ORM
- One-to-One Relationship
- One-to-Many Relationship
- Many to Many Eloquent Relationship
- Has One Through
- Has Many Through
- Querying Relations
- Middleware
- Laravel Views
- Blade Views
- Print data on view page
- Get Site URL
- Get URL Segment
- Get images from Storage folder
- Clear cache
- Form Class not found
- Flash Message in laravel
- Redirections
- path
- CRUD Projerct
- CRUD in Laravel
- CRUD progran
- Laravel Validation
- Jquery Validation
- Cookie
- Session
- Email Send in laravel
- File uploading
- CSRF Protection
- Helper in Laravel
- Helper Functions
- Guzzle Http Client
- Paypal Payment Gatway Integration
- Cron Job in laravel
- Flash message
- path
- Errors Handling
- Date Format
- Date Format Validation
- Display Image on View Page
- Update User Status using toggle button
- Delete Multiple Records using Checkbox in Laravel?
- Confirmation Before Delete Record
- Delete image from storage
- Remove/Trim Empty & Whitespace From Input Requests
- Block IP Addresses from Accessing Website
- How to Disable New User Registration in Laravel
- Redirect HTTP To HTTPS Using Laravel Middleware
- CKEditor
- slug generate unique
- Prevent Browser's Back Button After Logout
- Datatable dunamically
- encrypt & Decript
- Download File
- Rest API
- Shopping Cart
- Shopping Cart Example
- Dynamic Category-Subcategory Menu
- Ajax Search
- Interview Question
- laravel Tutorilal link
- laravel Tutorilal
Important Links
Laravel Routing
in In Laravel, all requests are outlined with the guidance of routes. All Laravel routes are determined in the file located as the app/Http/routes.php file, which is automatically stored and loaded by the framework. Primary routing named as basic routing routes the call may be referred as a request to the associated controllers.
Routing in Laravel includes the following categories −
Basic Routing
All Laravel routes are defined in the app/Http/routes.php file, which is automatically loaded by the framework. The most basic Laravel routes simply accept a URI and a Closure.
This file tells Laravel for the URIs it should respond to and the associated controller will give it a particular call.
Route::get('home', function () {
return 'Hello World';
});
Example
app/Http/routes.php
<?php
Route::get('/', function () {
return view('welcome');
});
when you simply run your laravel project url . it call the default url . and view the welcome page.
resources/view/welcome.blade.php
<html>
<head>
<title>Laravel</title>
<link href="https://fonts.googleapis.com/css?family=Lato:100" rel="stylesheet" type="text/css">
<style>
html, body {
height: 100%;
}
body {
margin: 0;
padding: 0;
width: 100%;
display: table;
font-weight: 100;
font-family: 'Lato';
}
.container {
text-align: center;
display: table-cell;
vertical-align: middle;
}
.content {
text-align: center;
display: inline-block;
}
.title {
font-size: 96px;
}
</style>
</head>
<body>
<div class="container">
<div class="content">
<div class="title">My Laravel 5</div>
</div>
</div>
</body>
</html>
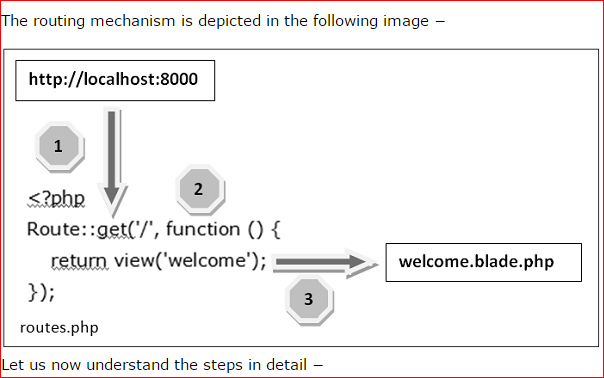
Step 1 − First, we need to execute the root URL of the application.
Step 2 − The executed URL will match with the appropriate method in the route.php file. In our case, it will match to get the method and the root (‘/’) URL. This will execute the related function.
Step 3 − The function calls the template file resources/views/welcome.blade.php. The function later calls the view() function with argument ‘welcome’ without using the blade.php. It will produce the following HTML output.
Sometimes you may need to register a route that responds to multiple HTTP verbs. You may do so using the match method. Or, you may even register a route that responds to all HTTP verbs using the any method:
Route::match(['get', 'post'], '/', function () {
//
});
Route::any('foo', function () {
//
});
Route Parameters :-
Required Parameters -
sometimes you will need to capture segments of the URI within your route. For example, you may need to capture a user's ID from the URL. You may do so by defining route parameters:
Route::get('user/{id}', function ($id) {
return 'User '.$id;
});
You may define as many route parameters as required by your route:
Route::get('posts/{post}/comments/{comment}', function ($postId, $commentId) {
//
});
Route parameters are always encased within "curly" braces. The parameters will be passed into your route's Closure when the route is executed.
Note: Route parameters cannot contain the - character. Use an underscore (_) instead.
Whatever argument that we pass after the root URL (http://localhost:8000/ID/5), it will be stored in $id and we can use that parameter for further processing but here we are simply displaying it. We can pass it onto view or controller for further processing.
Optional Parameters :-
Occasionally you may need to specify a route parameter, but make the presence of that route parameter optional. You may do so by placing a ? mark after the parameter name. Make sure to give the route's corresponding variable a default value:
Route::get('user/{name?}', function ($name = null) {
return $name;
});
Route::get('user/{name?}', function ($name = 'John') {
return $name;
});
There are some parameters which may or may not be present in the URL and in such cases we can use the optional parameters. The presence of these parameters is not necessary in the URL. These parameters are indicated by “?” sign after the name of the parameters. Here is the sample coding for routes.php file for that purpose.
Route::get('/user/{name?}',function($name = 'Virat'){
echo "Name: ".$name;
});
Example
routes.php
<?php
// First Route method – Root URL will match this method
Route::get('/', function () {
return view('welcome');
});
// Second Route method – Root URL with ID will match this method
Route::get('ID/{id}',function($id){
echo 'ID: '.$id;
});
// Third Route method – Root URL with or without name will match this method
Route::get('/user/{name?}',function($name = 'Virat Gandhi'){
echo "Name: ".$name;
});
Step 1 − Here, we have defined 3 routes with get methods for different purposes. If we execute the below URL then it will execute the first method.
http://localhost:8000
Step 2 − After successful execution of the URL, you will receive the following output −
Optional Parameters
Step 3 − If we execute the below URL, it will execute the 2nd method and the argument/parameter ID will be passed to the variable $id.
http://localhost:8000/ID/5
Step 4 − After successful execution of the URL, you will receive the following output −
ID 5
Step 5 − If we execute the below URL, it will execute the 3rd method and the optional argument/parameter name will be passed to the variable $name. The last argument ‘Virat’ is optional. If you remove it, the default name will be used that we have passed in the function as ‘Virat Gandhi’
http://localhost:8000/user/Virat
Step 6 − After successful execution of the URL, you will receive the following output −
Name Virat
Note − Regular expression can also be used to match the parameters.
Named Routes
Named routes allow a convenient way of creating routes. The chaining of routes can be specified using name method onto the route definition.
Route::get('user/profile', function () {
//
})->name('profile');
You may also specify route names for controller actions:
Route::get('user/profile', 'UserProfileController@showProfile')->name('profile');
The user controller will call for the function showProfile with parameter as profile. The parameters use name method onto the route definition.
Once you have assigned a name to your routes, you may use the route's name when generating URLs or redirects via the global route function:
// Generating URLs...
$url = route('profile');
// Generating Redirects...
return redirect()->route('profile');
which name is defined in the form action route name is similar to name to ruote.php name('same name');
example :
if you use route() method for link then you must specify the name in the route.php file .
<a href="{{route('show-link')}}"> Click Here</a>
<form action="{{route('myprofile')}}" method="post"/>
</form>
In Route.php file
Route::get('anything name here', 'UserProfileController@showProfile')->name('show-link');
Route::post('anything name here', 'UserProfileController@showProfile')->name('myprofile');
Create Simple Route:
Route::get('myroute', function () {
return 'This is Simple Route Example of itechxpert';
});
http://localhost:8000/myroute
Route with Call View File:
Route::view('my-route', 'index');
resources/views/index.php
his is Simple Route Example of itechxpert
Access URL:
http://localhost:8000/my-route
Route with Controller Method:
Route::get('my-route', 'TestController@index');
Create Route with Parameter:
simple route with passing parameters.
Route::get('users/{id}', 'UserController@show');
class UserController extends Controller
{
/**
* Show the application dashboard.
*
* @return \Illuminate\Contracts\Support\Renderable
*/
public function show($id)
{
return 'User ID:'. $id;
}
}
Access URL:
http://localhost:8000/users/21
Create Route Methods:
You can create get, post, delete, put, patch methods route
Route::get('users', 'UserController@index');
Route::post('users', 'UserController@post');
Route::put('users/{id}', 'UserController@update');
Route::delete('users/{id}', 'UserController@delete');
How to get current route name in Laravel
return \Request::route()->getName(); // response productCRUD.index
return \Route::currentRouteName(); // response productCRUD.index
How to get current route path in Laravel
return \Route::getCurrentRoute()->getPath(); // response productCRUD
How to get current route action in Laravel
return \Route::getCurrentRoute()->getActionName();
How to get a list of all routes in Laravel?
$routes = \Route::getRoutes();
foreach ($routes as $route) {
echo $route->getPath().'
';
}
How to Get Current Route Name
$routeName = Request::route()->getName());
print($routeName);
$routeName = Route::getCurrentRoute()->getPath();
print($routeName);
How to pass route in href tag
In your route put name and
Route::get('/dashboard', function () {
return view('admin.dashboard')->name(dashboard);
});
Go to your html where you link the url
<a id="index" class="navbar-brand" href="{{route('dashboard')}}">
<img src="../img/itechxpert.png" alt="Logo">
</a>
if you use route in href tag then you must define route name in your routing file.
How can I pass parameter in route href
know more
Route::get('/test/{id}', 'HomeController@test')->name('test');
How To Get Route Name In lavel Controller?
public function index()
{
$route_Name = Request::route()->getName();
dd($route_Name);
$routeName = Route::currentRouteName();
dd($routeName);
$routeName = Route::current();
dd($routeName->action['as']);
}
How to Generate Url From Name Routes in laravel?
Route::get('home', 'HomeController@index')->name('home');
Simple url To route
Simple Parameter Url To Routes
Route::get('posts/{id}', 'PostsController@show')->name('post');
Link to Resource {{ $id }}
Adding Additional Parameters
Route::get('posts/{post}/comments/{comment}', 'CommentController@show')->name('commentshow');
Comment show
Routing
<?php
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('index');
});
//Route::get('/aboutus', function () { return view('aboutus');});
Route::get('about-us','frontend\AboutusController@index')->name('about');
/*Route::get('/contact', function () {
return view('contactus');
}); */
Route::get('courses','frontend\CoursesController@index')->name('courses');
Route::get('teacher','frontend\TeacherController@index')->name('teacher');
Route::get('blog','frontend\BlogController@index')->name('blog');
Route::get('contact-us','frontend\ContactUsController@index')->name('contact');
Route::post('contact-me','frontend\ContactUsController@contact_me');
//Route::post('contact-me', 'frontend\EmailController@sendEmail');
Route::get('/admin', function () {
return view('auth.login');
});
Auth::routes();
// for email verify
Auth::routes(['verify' => true]);
Route::get('/home', 'admin\HomeController@index')->name('home');
Route::get('dashboard', 'admin\DashboardController@index');
Route::get('logout', 'Auth\LoginController@logout')->name('logout');
Route::get('reset-password', 'Auth\ResetPasswordController@create')->name('reset-password');
Route::post('password-update', 'Auth\ResetPasswordController@store')->name('password.update');
Route::get('logo-name', 'admin\HomeController@add_logo_name')->name('logo_name');
Route::post('logoadd', 'admin\HomeController@add_logo')->name('logoadd');
Route::get('slider', 'admin\HomeController@slider_show')->name('slider');
Route::post('addslider', 'admin\HomeController@slider_add');
//Route::get('users', 'admin\HomeController@index');
Route::get('sliderChangeStatus', 'admin\HomeController@sliderChangeStatus');
/* ################# Category Route ##################### */
Route::get('add-category', 'admin\CategoryController@add_category');
Route::post('category-add', 'admin\CategoryController@category_save')->name('addcategory');
Route::get('category-list', 'admin\CategoryController@category_show')->name('categorylist');
Route::post('addcategorymodal', 'admin\CategoryController@category_add');
Route::get('categories','admin\CategoryController@category_subcategory');
Route::get('categories/{id}','admin\CategoryController@getCategory')->name('categories');
/* ################# End Category Route ##################### */
Route::get('aboutus', 'admin\AboutusController@index');
Route::post('add-aboutus', 'admin\AboutusController@add_aboutus')->name('aboutus_add');
Route::get('showaboutus', 'admin\AboutusController@show');
Route::get('aboutusdelete', 'admin\AboutusController@delete_aboutus');
Route::get('edit-aboutus/{id}/edit', 'admin\AboutusController@editaboutus');
Route::post('update-aboutus', 'admin\AboutusController@update_aboutus');
Route::post('addaboutmodel', 'admin\AboutusController@add_aboutus_model');
Route::get('aboutus/edit/{id}', 'admin\AboutusController@aboutuspage_edit');
Route::post('aboutus_update/{id}', 'admin\AboutusController@aboutuspage_update');
Route::get('gallery', 'admin\GalleryController@index');
Route::post('add-gallery', 'admin\GalleryController@add_gallery')->name('gallery_add');
Route::get('showgallery', 'admin\GalleryController@show');
Route::get('galleryimagedelete', 'admin\GalleryController@galleryimagedelete');
Route::get('edit-gallery/{id}/edit', 'admin\GalleryController@editgallery');
Route::post('update-gallery', 'admin\GalleryController@update_gallery');
Route::post('update-gallery_ajax/{id}/update', 'admin\GalleryController@update_gallery_ajax');
Route::post('gallerydel/{id}','admin\GalleryController@destroy')->name('gallery.destroy');
Route::get('/status/update', 'admin\GalleryController@updateStatus')->name('gallery.update.status');
Route::get('resizeImage', 'ImageController@resizeImage');
Route::post('resizeImagePost', 'ImageController@resizeImagePost')->name('resizeImagePost');
Route::get('imageuploadpreview','admin\GalleryController@image_preview');
Route::post('save-photo', 'admin\GalleryController@saveimage');
Route::get('contactus', 'admin\ContactusController@index');
Route::post('add-contactus', 'admin\ContactusController@add_contactus')->name('contactus_add');
Route::get('showcontactus', 'admin\ContactusController@show');
Route::get('contactusdelete', 'admin\ContactusController@delete_contactus');
Route::get('edit-contactus/{id}/edit', 'admin\ContactusController@editcontactus');
Route::post('update-contactus', 'admin\ContactusController@update_contactus');
Route::get('service', 'admin\ServicesController@index');
Route::post('service-add', 'admin\ServicesController@service_add')->name('service_add');
Route::get('serviceshow', 'admin\ServicesController@serviceshow');
Route::get('blogs', 'admin\BlogController@index');
Route::post('blog-add', 'admin\BlogController@blog_add')->name('blog_add');
Route::get('blogshow', 'admin\BlogController@blogshow');
Route::get('blankpage', 'admin\HomeController@blankpage')->name('samplepage');
/* ############# Relationship ###################### */
Route::get('add-product', 'admin\ProductController@index')->name('addproduct');
Route::post('product-add', 'admin\ProductController@product_add')->name('product_add');
Route::get('product-show', 'admin\ProductController@show_products')->name('showproduct');
Route::get('viewproduct/{id}', 'admin\ProductController@viewbyid');
Route::get('addattribute/{id}', 'admin\ProductController@add_attribute');
Route::get('deleteproduct/{id}', 'admin\ProductController@delete_product');
Route::get('add-company', 'admin\CompanyController@index')->name('addcompany');
Route::post('company-add', 'admin\CompanyController@company_add')->name('company_add');
Route::get('show-company', 'admin\CompanyController@show_car')->name('showcompany');
Route::get('viewcompany/{id}', 'admin\CompanyController@viewcompany');
Route::get('many-to-many', 'admin\ManytoManyController@index')->name('manytomany');
Route::get('show-manytomany', 'admin\ManytoManyController@show_many_to_many')->name('showmanytomany');
/* ############# End Relationship ###################### */
/* #################### Student API #################### */
Route::get('student-add', 'admin\ApiController@index');
Route::get('students', 'admin\ApiController@getAllStudents');
Route::post('studentsave', 'admin\ApiController@createStudent');
Route::get('students/{id}', 'admin\ApiController@getStudent');
Route::put('students/{id}', 'admin\ApiController@updateStudent');
Route::delete('students/{id}','admin\ApiController@deleteStudent');
/* #################### End Student API #################### */
Route::get('language','admin\LanguageController@index');
Route::post('language','admin\LanguageController@store')->name('language.store');
Route::get('multiselectdropdown','admin\MultiselectController@create');
Route::post('multiselect-dropdown','admin\MultiselectController@store')->name('multiselectdropdown');
Route::get('toster','admin\HomeController@tostercreate');
Route::get('menus','admin\MenuController@index');
Route::get('menus-show','admin\MenuController@show');
Route::post('menus','admin\MenuController@store')->name('menus.store');
Route::get('selectoption','admin\DemoController@index');
Route::post('addselect','admin\DemoController@add_select');
Route::get('modalpopup-validation','admin\ModalpopupController@index');
Route::post('registeruser','admin\ModalpopupController@store');
Route::get('uploadfile-progressbar','admin\UploadFileProgressController@index');
Route::get('passwordhash','admin\UserPasswordController@index');
Route::post('passwordverify','admin\UserPasswordController@verify_password');