C Tutorials
- What is C?
- History Of C?
- Feature of C?
- How to Install C?
- How to write c program?
- First c program
- Flow of C program
- printf() and scanf() in C
- C Program Structure
- Variables in C
- Data Types in C
- Keywords in C
- C Operators
- Comments in C
- Escape Sequence in C
- Escape Sequence in C
- Constants in C
- C - Storage Classes
- C - Decision Making
- Switch statement in C
- C Loops
- Loop Control Statements
- Type Casting in C
- functions in C
- call by value Or call by reference
- Recursion in C
- Storage Classes in C
- Array
- String
- Pointer
- Pointer And Array
- Pointer with function
- Structure
- Union
- File Handling
- Preprocessor Directives
Important Links
C - Decision Making
The Decision making statement checks the given condition and then executes its subblock. The conditional statements use relational operators. Decision making is about deciding the order of execution of statements based on certain conditions or repeat a group of statements until certain specified conditions are met.
Following statements are known as controller decision making statements.
Decision making with if statement
The if statement may be implemented in different forms depending on the complexity of conditions to be tested. The different forms are,
if statement:-
The statement is executed only when the condition is true. if the condition is false the compiler skips the lines within the if block. the condition is always enclosed within a pair of parenthesis. The conditional statement should not be terminated with semicolon. The if statement are normally enclosed with curly braces.
if(expression)
{
statement inside;
}
statement outside;
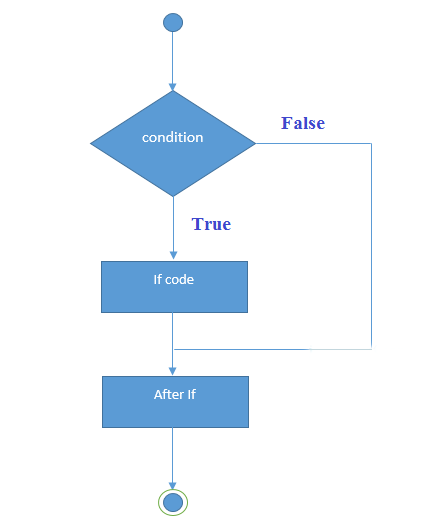
Example :-
#include<stdio.h>
int main(){
int number=0;
printf("enter a number:");
scanf("%d",&number);
if(number%2==0){
printf("%d is even number",number);
}
return 0;
}
Output :-
4 is even number
Example :-
#include<stdio.h>
void main( )
{
int x, y;
x = 15;
y = 13;
if (x > y )
{
printf("x is greater than y");
}
}
If-else Statement
The if..else statement takes care of true as well as false conditions. it has two blocks. first block is executed when the condition is true. the other block is of else and it is executed when the condition is false. the else statement cannot be used without if.
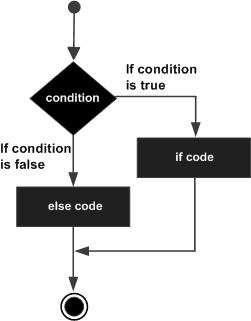
if(expression)
{
statement block1;
}
else
{
statement block2;
}
statement block3;
If the expression is true, the statement-block1 is executed, else statement-block1 is skipped and statement-block2 is executed.
Example :-
#include<stdio.h>
void main( )
{
int x, y;
x = 15;
y = 18;
if (x > y )
{
printf("x is greater than y");
}
else
{
printf("y is greater than x");
}
}
Example :-
#include<stdio.h>
int main () {
/* local variable definition */
int a = 100;
/* check the boolean condition */
if( a < 20 ) {
/* if condition is true then print the following */
printf("a is less than 20\n" );
} else {
/* if condition is false then print the following */
printf("a is not less than 20\n" );
}
printf("value of a is : %d\n", a);
return 0;
}
Nested if....else statement
if( expression )
{
if( expression1 )
{
statement block1;
}
else
{
statement block2;
}
}
else
{
statement block3;
}
void main( )
{
int a, b, c;
printf("Enter 3 numbers...");
scanf("%d%d%d",&a, &b, &c
if(a > b)
{
if(a > c)
{
printf("a is the greatest");
}
else
{
printf("c is the greatest");
}
}
else
{
if(b > c)
{
printf("b is the greatest");
}
else
{
printf("c is the greatest");
}
}
}
else if ladder
if(expression1)
{
statement block1;
}
else if(expression2)
{
statement block2;
}
else if(expression3 )
{
statement block3;
}
else
default statement;
The expression is tested from the top(of the ladder) downwards. As soon as a true condition is found, the statement associated with it is executed.
Example :-
#include<stdio.h>
void main( )
{
int a;
printf("Enter a number...");
scanf("%d", &a);
if(a%5 == 0 && a%8 == 0)
{
printf("Divisible by both 5 and 8");
}
else if(a%8 == 0)
{
printf("Divisible by 8");
}
else if(a%5 == 0)
{
printf("Divisible by 5");
}
else
{
printf("Divisible by none");
}
}
Points to Remember :-
- In
if
statement, a single statement can be included without enclosing it into curly braces{ ... }
int a = 5; if(a > 4) printf("success");
No curly braces are required in the above case, but if we have more than one statement inside
if
condition, then we must enclose them inside curly braces. ==
must be used for comparison in the expression ofif
condition, if you use=
the expression will always return true, because it performs assignment not comparison.- Other than 0(zero), all other values are considered as true.
if(27) printf("hello");
In above example, hello will be printed.