C Tutorials
- What is C?
- History Of C?
- Feature of C?
- How to Install C?
- How to write c program?
- First c program
- Flow of C program
- printf() and scanf() in C
- C Program Structure
- Variables in C
- Data Types in C
- Keywords in C
- C Operators
- Comments in C
- Escape Sequence in C
- Escape Sequence in C
- Constants in C
- C - Storage Classes
- C - Decision Making
- Switch statement in C
- C Loops
- Loop Control Statements
- Type Casting in C
- functions in C
- call by value Or call by reference
- Recursion in C
- Storage Classes in C
- Array
- String
- Pointer
- Pointer And Array
- Pointer with function
- Structure
- Union
- File Handling
- Preprocessor Directives
Important Links
Loop Control Statements
Sometimes, while executing a loop, it becomes necessary to skip a part of the loop or to leave the loop as soon as certain condition becomes true. This is known as jumping out of loop.
break statement :-
The break statement in C programming has the following two usages −
When a break statement is encountered inside a loop, the loop is immediately terminated and the program control resumes at the next statement following the loop.
It can be used to terminate a case in the switch statement
If you are using nested loops, the break statement will stop the execution of the innermost loop and start executing the next line of code after the block.
flow :-
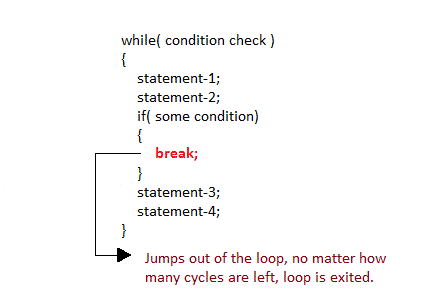
int main () {
/* local variable definition */
int a = 10;
/* while loop execution */
while( a < 20 ) {
printf("value of a: %d\n", a);
a++;
if( a > 15) {
/* terminate the loop using break statement */
break;
}
}
return 0;
}
int main(){
int i=1;//initializing a local variable
//starting a loop from 1 to 10
for(i=1;i<=10;i++){
printf("%d \n",i);
if(i==5){//if value of i is equal to 5, it will break the loop
break;
}
}
return 0;
}
continue statement :-
The continue statement in C language is used to continue the execution of loop (while, do while and for). It is used with if condition within the loop.
In case of inner loops, it continues the control of inner loop only.
It causes the control to go directly to the test-condition and then continue the loop process. On encountering continue, cursor leave the current cycle of loop, and starts with the next cycle.
In case of inner loops, it continues the control of inner loop only.
It causes the control to go directly to the test-condition and then continue the loop process. On encountering continue, cursor leave the current cycle of loop, and starts with the next cycle.
flow :-
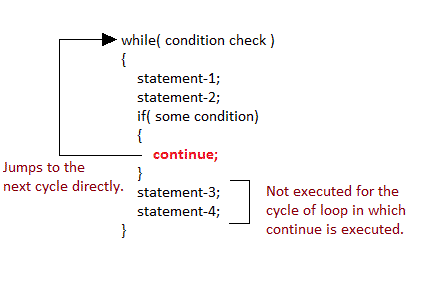
int main () {
/* local variable definition */
int a = 10;
/* do loop execution */
do {
if( a == 15) {
/* skip the iteration */
a = a + 1;
continue;
}
printf("value of a: %d\n", a);
a++;
} while( a < 20 );
return 0;
}
int main(){
int i=1;//initializing a local variable
//starting a loop from 1 to 10
for(i=1;i<=10;i++){
if(i==5){//if value of i is equal to 5, it will continue the loop
continue;
}
printf("%d \n",i);
}//end of for loop
return 0;
}
Output :-
1
2
3
4
6
7
8
9
10
As you can see, 5 is not printed on the console because loop is continued at i==5.
As you can see, 5 is not printed on the console because loop is continued at i==5.
goto statement :-
The goto statement is known as jump statement in C language. It is used to unconditionally jump to other label. It transfers control to other parts of the program.
It is rarely used today because it makes program less readable and complex.
It is rarely used today because it makes program less readable and complex.
int main() {
int age;
ineligible:
printf("You are not eligible to vote!\n");
printf("Enter you age:\n");
scanf("%d", &age);
if(age<18)
goto ineligible;
else
printf("You are eligible to vote!\n");
return 0;
}
int main () {
/* local variable definition */
int a = 10;
/* do loop execution */
LOOP:do {
if( a == 15) {
/* skip the iteration */
a = a + 1;
goto LOOP;
}
printf("value of a: %d\n", a);
a++;
}while( a < 20 );
return 0;
}