C Tutorials
- What is C?
- History Of C?
- Feature of C?
- How to Install C?
- How to write c program?
- First c program
- Flow of C program
- printf() and scanf() in C
- C Program Structure
- Variables in C
- Data Types in C
- Keywords in C
- C Operators
- Comments in C
- Escape Sequence in C
- Escape Sequence in C
- Constants in C
- C - Storage Classes
- C - Decision Making
- Switch statement in C
- C Loops
- Loop Control Statements
- Type Casting in C
- functions in C
- call by value Or call by reference
- Recursion in C
- Storage Classes in C
- Array
- String
- Pointer
- Pointer And Array
- Pointer with function
- Structure
- Union
- File Handling
- Preprocessor Directives
Important Links
WHAT IS C FUNCTION?
A function is a block of statements that performs a specific task.
C function contains set of instructions enclosed by “{ }” which performs specific operation in a C program.
The function in C language is also known as procedure or subroutine in other programming languages.
A function can be called many times. It provides modularity and code reusability.
There are many situations where we might need to write same line of code for more than once in a program. This may lead to unnecessary repetition of code, bugs and even becomes boring for the programmer. So, C language provides an approach in which you can declare and define a group of statements once in the form of a function and it can be called and used whenever required.
C program has at least one function, which is main().
USES OF C FUNCTIONS: :-
Functions are used because of following reasons –
Types of Functions :-
Library functions are those functions which are already defined in C library, example printf(), scanf(), strcat() etc. You just need to include appropriate header files to use these functions. These are already declared and defined in C libraries.
A User-defined functions are those functions which are defined by the user at the time of writing program. These functions are made for code reusability and for saving time and space.
Declaration of a function :-
body of the function
}
a function must also be declared before its used. Function declaration informs the compiler about the function name, parameters is accept, and its return type. The actual body of the function can be defined separately. It's also called as Function Prototyping.
returntype :-
A function may return a value. The return_type is the data type (int, float, char, double) of the value the function returns. Some functions perform the desired operations without returning a value. In this case, the return_type is the keyword void.
A C function may or may not return a value from the function. If you don't have to return any value from the function, use void for the return type.
functionName :-
Function name is an identifier and it specifies the name of the function. The function name is any valid C identifier.
The functionName is name of the function to be called.
parameter list :-
The parameter list declares the type and number of arguments that the function expects when it is called. Also, the parameters in the parameter list receives the argument values when the function is called.
Argument list contains variables names along with their data types. These arguments are kind of inputs for the function. For example – A function which is used to add two integer variables, will be having two integer argument.
Function Body :-
The function body contains a collection of statements that define what the function does.
Example of C function with no return statement
#include<stdio.h>
void show(){
printf("Hello itechxpert \n");
}
int main(){
show();//calling a function
show();
return 0;
}
Example of C function with return statement
#include<stdio.h>
//defining function
int cube(int n){
return n*n*n;
}
int main(){
int result1=0,result2=0;
result1=cube(2);//calling function
result2=cube(3);
printf("%d \n",result1);
printf("%d \n",result2);
return 0;
}
Output :-
27
#include<stdio.h>
int multiply(int a, int b); // function declaration
int main()
{
int i, j, result;
printf("Please enter 2 numbers you want to multiply...");
scanf("%d%d", &i, &j);
result = multiply(i, j); // function call
printf("The result of muliplication is: %d", result);
return 0;
}
int multiply(int a, int b)
{
return (a*b); // function defintion, this can be done in one line
}
Passing Arguments to a function :-
Arguments are the values specified during the function call, for which the formal parameters are declared while defining the function.
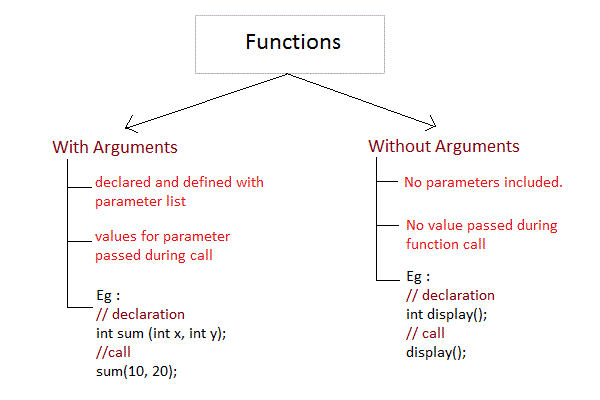
It is possible to have a function with parameters but no return type. It is not necessary, that if a function accepts parameter(s), it must return a result too.
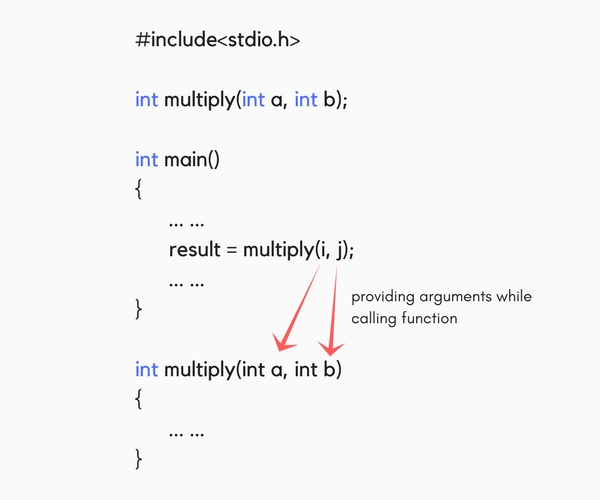
While declaring the function, we have declared two parameters a and b of type int. Therefore, while calling that function, we need to pass two arguments, else we will get compilation error. And the two arguments passed should be received in the function definition, which means that the function header in the function definition should have the two parameters to hold the argument values. These received arguments are also known as formal parameters. The name of the variables while declaring, calling and defining a function can be different.
Returning a value from function :-
A function may or may not return a result. But if it does, we must use the return statement to output the result. return statement also ends the function execution, hence it must be the last statement of any function. If you write any statement after the return statement, it won't be executed.
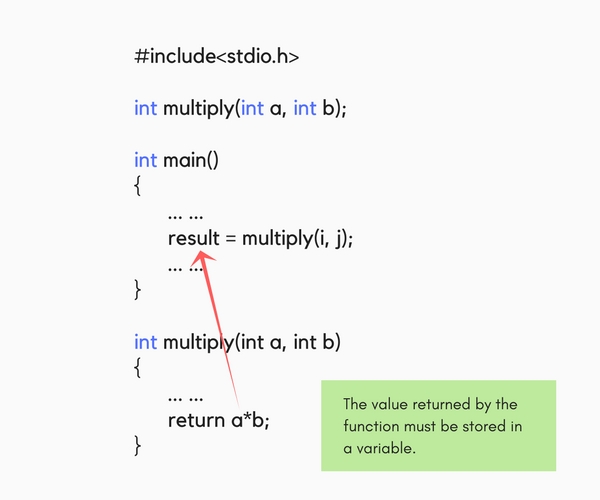
The datatype of the value returned using the return statement should be same as the return type mentioned at function declaration and definition. If any of it mismatches, you will get compilation error.
#include<stdio.h>
// function prototype, also called function declaration
float square ( float x );
// main function, program starts from here
int main( )
{
float m, n ;
printf ( "\nEnter some number for finding square \n");
scanf ( "%f", &m ) ;
// function call
n = square ( m ) ;
printf ( "\nSquare of the given number %f is %f",m,n );
}
float square ( float x ) // function definition
{
float p ;
p = x * x ;
return ( p ) ;
}